Extract text using regular expressions
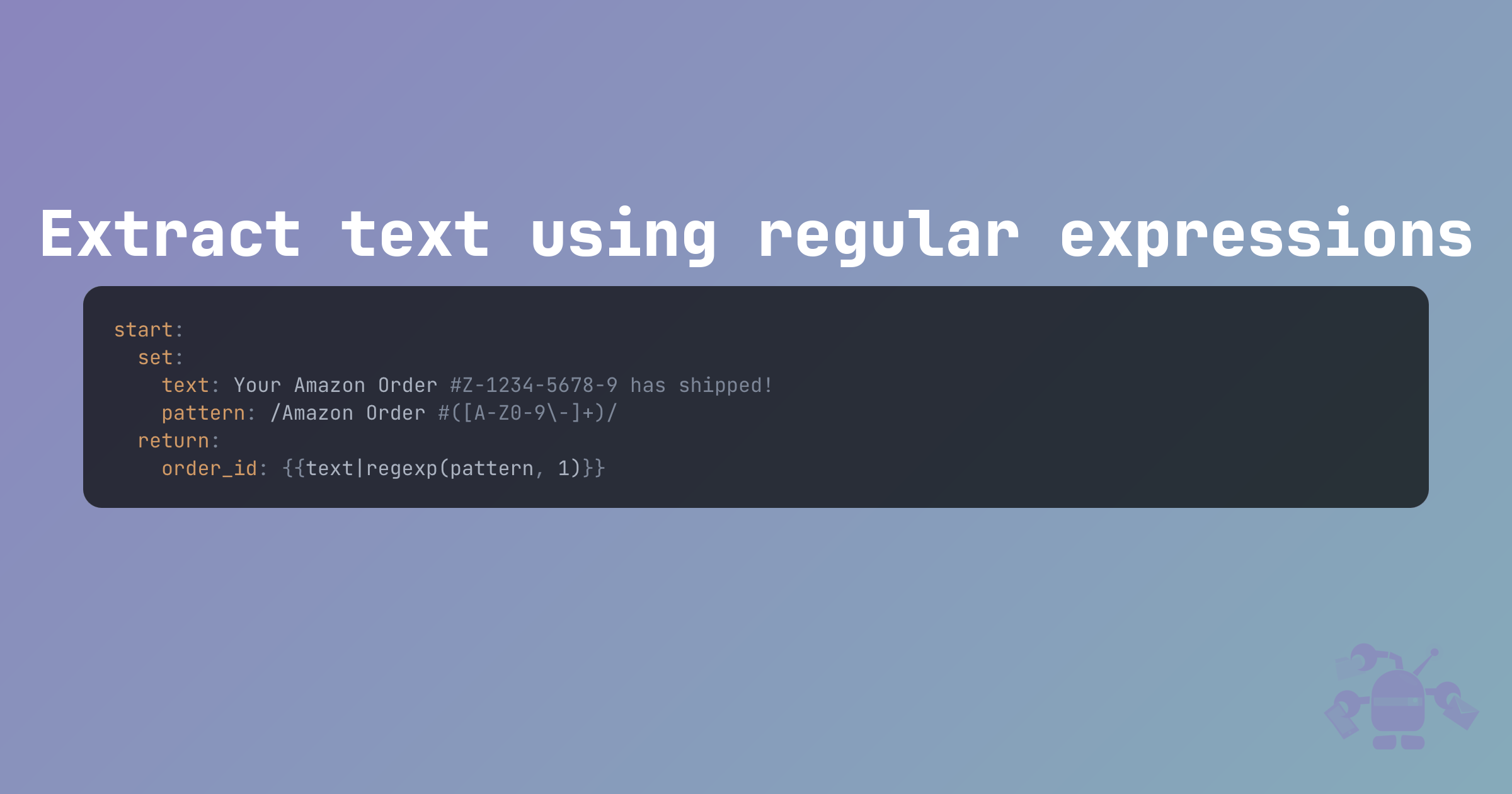
Here are examples of using regular expressions to extract matching text in automation scripting.
Matching a single capture group
The pattern is a KATA key.
start:
set:
text: Your Amazon Order #Z-1234-5678-9 has shipped!
pattern: /Amazon Order #([A-Z0-9\-]+)/
return:
order_id: {{text|regexp(pattern, 1)}}
Setting the pattern as a variable
The pattern is a scripting variable.
start:
set:
mask@text:
{% set text = "The ticket mask that I am looking for is: KRN-69622-357 something else" %}
{% set pattern %}/[A-Z]{3}-\d{5}-\d{3}/{% endset %}
{{text|regexp(pattern)}}
outcome/hasMask:
if@bool: {{mask}}
then:
return:
output: The ticket mask is #: {{mask}}
Using multiple capture groups
The second argument to |regexp
specifies the capture group to return.
start:
set:
text: (123,456)
pattern: /^\((\d+),(\d+)\)$/
return:
x@int: {{text|regexp(pattern, 1)}}
y@int: {{text|regexp(pattern, 2)}}
Returning all matches for all capture groups
Use the regexp_match_all() function to return multiple capture groups for all matches.
-
start: set: headers@text: X-Mailer: Cerb From: customer@cerb.example To: support@cerb.example return: results@text: {% set results = regexp_match_all("#^(.*?): (.*?)$#m", headers) %} {{results|json_encode|json_pretty}}
-
__return: results: |- [ [ "X-Mailer: Cerb", "From: customer@cerb.example", "To: support@cerb.example" ], [ "X-Mailer", "From", "To" ], [ "Cerb", "customer@cerb.example", "support@cerb.example" ] ]